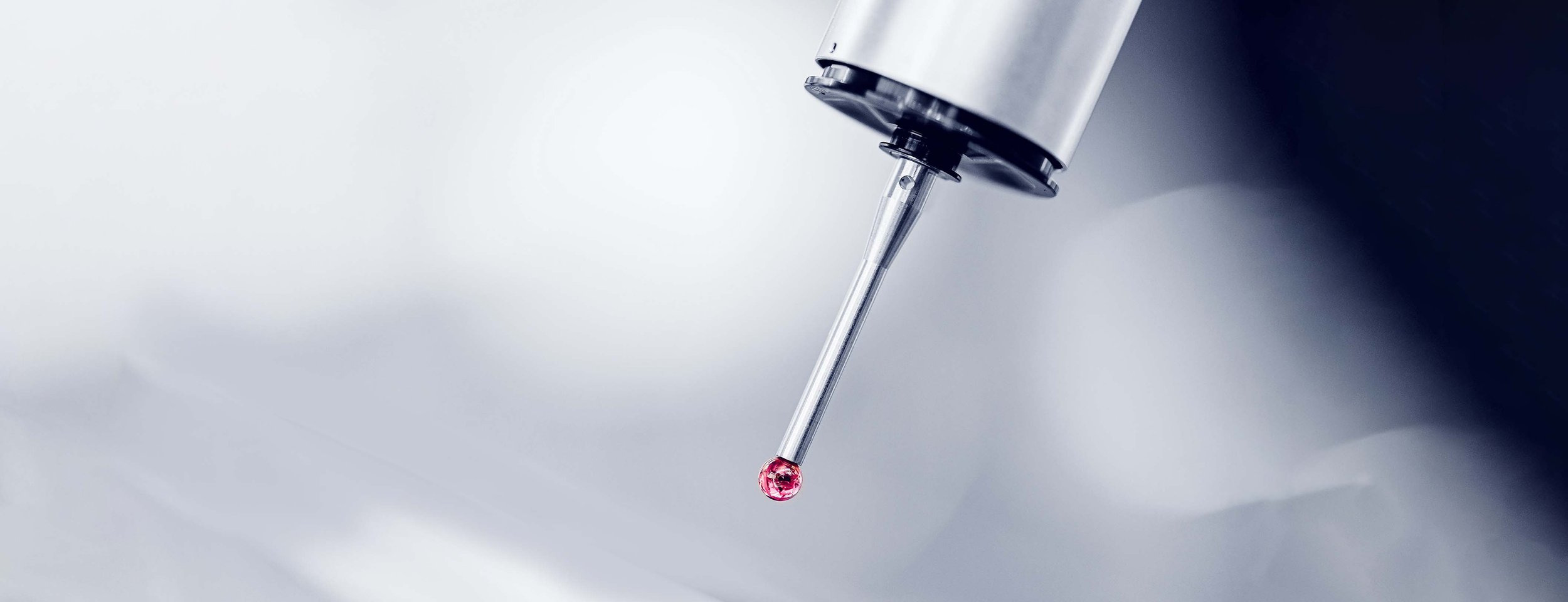
Advanced Macro Programming
This article covers the following:
The G65 program call
Creating custom G codes
Advanced Probing
G65 macro program call
This article continues where the Intro to Macro Programming article left off. To continue we’re going to look at the G65 sub program macro call. In the previous article, we had just written the code to peel mill a slot with an end mill allowing variables such as stepover, end mill size, slot size and entry/exit radius to be changed. Here is the diagram and code once again in Figure 1:
Figure 1: Peel milling example previously used in Intro to Macro Programming
#100=0.5 (End mill size variable)
#110=0.05 (Stepover)
#111=1.5 (Slot width)
#101= [#111/2]-[#100/2] (No 5 Y position calculation)
#102=[-[#100/2]+#110] (No 3 X position calculation)
#103=0.1*#100 (Enter and Exit radius)
G00 X[#102-#103-0.1] Y-#101 (Position 1)
Z.1
G01 Z-1.5
WHILE [[#102-#103] LE 3] DO1
G01 X[#102-#103] (Position 2)
G03 X#102 Y[-#101+#103] R#103 (Position 3)
G01 Y[#101-#103] (Position 4)
G03 X[#102-#103] Y#101 R#103 (Position 5)
G00 Y-#101 (Back to Position 2)
#102=#102+#110 (Stepover)
END1
G65 allows us to call a macro subprogram and pass it variables. So far we’ve only used macro commands within a program and changed variables accordingly. Using the G65 call allows us to pass values to a stand alone macro program which makes the program on the screen clear and concise. G65 follows the following format:
G65 P9000 S0.05 D0.5 W1.5
Here G65 is calling macro subprogram O9000 and passing it arguments S, D, and W. The program number is really just another program with O9000 as the number. The G65 call differs from M98 sub program call because of the ability to pass the subprogram new information in the form of arguments which are S0.05, D0.5, and W1.5 in our example. You’ll notice the arguments are letters in the G65 call but all the variables we’ve used in calculations so far are numbers. When the G65 program calls O9000 the letters S, D, and W are converted to numbers according to the following chart:
Argument | Variable |
---|---|
A | #1 |
B | #2 |
C | #3 |
D | #7 |
E | #8 |
F | #9 |
H | #11 |
I | #4 |
J | #5 |
K | #6 |
M | #13 |
Q | #17 |
R | #18 |
S | #19 |
T | #20 |
U | #21 |
V | #22 |
W | #23 |
X | #24 |
Y | #25 |
Z | #26 |
Again, when using our example macro call: G65 P9000 S0.05 D0.5 W1.5, the variables #19 (the letter S), #7 (the letter D) and #23 (letter W), are populated in the control memory and read by our macro subprogram O9000. So when we use the variables, #19, #7, and #23 in the 09000 program they will already have the values of 0.05, 0.5, and 1.5 , respectively if called by G65.
So now let’s rewrite the macro above to be able to use the variables for stepover (letter S and #19), end mill size (letter D and #7) and slot size (letter W and #23). We’ll be making the following substitutions to allow G65 usage:
#100 substituted with #7 (End mill size)
#110 substituted with #19 (Stepover)
#111 substituted with #23 (Slot width)
We’ll also be taking out the first three lines which used to define what the end mill size, stepover, and slot width and since the G65 call is defining them.
%
09000
#101= [#23/2]-[#7/2] (No 5 Y position calculation)
#102=[-[#7/2]+#19] (No 3 X position calculation)
#103=0.1*#7 (Enter and Exit radius)
G00 X[#102-#103-0.1] Y-#101 (Position 1)
Z.1
G01 Z-1.5
WHILE [[#102-#103] LE 3] DO1
G01 X[#102-#103] (Position 2)
G03 X#102 Y[-#101+#103] R#103 (Position 3)
G01 Y[#101-#103] (Position 4)
G03 X[#102-#103] Y#101 R#103 (Position 5)
G00 Y-#101 (Back to Position 2)
#102=#102+#19 (Stepover)
END1
M99
%
Note the M99 at the end to escape the macro program and return to the main program. The main program would look something like this:
%
O0001
T1 H1 G43 M06
S2000 M03
G00 X0 Y0
G65 P9000 S0.05 D0.5 W1.5
G00 Z1.
G28 G91 Z0 Y0
G90
M30
%
This is much cleaner than the many lines of code we started with, easier to read and not as prone to typos or copy and paste errors. If you save the macro program in the machine file directory, you’ll be able to call it at any time. Please note you have to save the macro program as a 8000 or 9000 series program to use G65. The main program uses the standard program numbers O0001 - O7999.
Custom G codes
Creating custom G codes or G code aliasing as it’s also known, will eliminate the need to write the code G65 P9000 S0.05 D0.5 W1.5 and instead look a like this: G194 S0.05 D0.5 W1.5 which is just like the standard G codes that Fanuc and HAAS have created (G194 here is just a random example). All it takes is linking the G code to the macro program number in the parameter settings of your control.
For HAAS parameters 91-100 alias the G codes you choose with macro programs O9010-O9019:
Macro Program | HAAS Parameter |
---|---|
O9010 | 91 |
O9011 | 92 |
O9012 | 93 |
O9013 | 94 |
O9014 | 95 |
O9015 | 96 |
O9016 | 97 |
O9017 | 98 |
O9018 | 99 |
O9019 | 100 |
For example, if we set parameter 98 to 111 it would make G111 equivalent to G65 P9017
Fanuc also has it’s own G code parameters:
Macro Program | FANUC Parameter |
---|---|
O9010 | 6050 |
O9011 | 6051 |
O9012 | 6052 |
O9013 | 6053 |
O9014 | 6054 |
O9015 | 6055 |
O9016 | 6056 |
O9017 | 6057 |
O9018 | 6058 |
O9019 | 6059 |
Advanced Probing
Your machine tool probe likely came with software that allows you to run many standard probing cycles such as X, Y, and Z surface measurements, bore measurements, and web/pocket measurements. Most of these cycles are used to set work shift values, but you can also use your probe to measure and use the measured values as inputs in your program which we’ll cover here. For example, in our slot program above, we can machine the slot, then use a probe to measure the depth of the slot, write some macro code to decide if it’s to spec and rerun the program where we reposition the tool, if necessary. The following will focus on HAAS machine tools and Renishaw parameters and variables only.
Probe Variables
The probe saves information it collects in variables and should be available information from the probe manufacturer or machine tool manufacturer. Here’s a small sample of some popular probe cycles and variables where measurements are saved:
Cycle G65 P9811, single surface, saves the the X position in variable #185, Y position in #186 and Z position in #187
Cycle G65 P9812, web or pocket, saves the the X position in variable #185 and Y position in #186
Cycle G65 P9814, bore or boss, saves the the X position in variable #185 and Y position in #186
For example, when you probe the Z position using the following code, the value will be saved in #187:
T25 H25 G43 M06 (call probe)
G00 X1. Y0
Z5.
G65 P9832 (probe on)
G65 P9810 Z-1.0 F10. (protected positioning move to Z-1.0)
G65 P9811 Z-1.5 Q0.7 (Z position, single surface measurement at Z-1.5, note there is no work shift altering)
G65 P9833 (probe off)
G91 G28 Z0 (send tool home)
G90
After this is run, let’s just assume the #187 value is -1.495, instead of -1.500 which we are targeting, which is off by 0.005". Assume we have a size and tolerance of -1.500 +/-0.001, so we write in some logic to allow the control to decide if the part passes, is scrap or is shallow and needs to be rerun (I’m ignoring any tool deflection during cutting for the sake of this example):
IF[#187LT-1.501] GOTO500 (check if the tool already cut deeper than 1.501” and alarm)
#600=-1.5-(#187) (calculate the difference between measured (#187) and ideal (1.500) depth and save in variable #600).
IF[#187GT-1.499] GOTO100 (if the tool cut shallower than 1.499, go to the beginning of the program, N100, and recut)
N500 #3000=100(Tool cut too deep) (alarm)
Here we first check if the tool has already cut too deep, in which case we simply code in an alarm. If the tool hasn’t cut too deep, we calculate the difference and save in variable #600. We then check if the tool cut too shallow and move to the beginning of the program.
Let’s now complete our slot milling macro program to include the probing and re-machining. We now need to alter our original code to do the following (colors correspond to code):
initialize #600=0 and #187 to zero
add the end mill tool call/retract with spindle speed and feed in the macro program
add the variable #600 in the depth positioning
add the probing code from above
add the logic depth check
%
O9000
#600=0
#187=0
N100
T01 H01 G43 M06
S2000 F50. M03
#101= [#23/2]-[#7/2] (No 5 Y position calculation)
#102=[-[#7/2]+#19] (No 3 X position calculation)
#103=0.1*#7 (Enter and Exit radius)
G00 X[#102-#103-0.1] Y-#101 (Position 1)
Z.1
G01 Z[-1.5+#600]
WHILE [[#102-#103] LE 3] DO1
G01 X[#102-#103] (Position 2)
G03 X#102 Y[-#101+#103] R#103 (Position 3)
G01 Y[#101-#103] (Position 4)
G03 X[#102-#103] Y#101 R#103 (Position 5)
G00 Y-#101 (Back to Position 2)
#102=#102+#19 (Stepover)
END1
G91 G28 Z0 (send tool home)
G90
T25 H25 G43 M06 (probe call)
G00 X1. Y0
Z5.
G65 P9832 (probe on)
G65 P9810 Z-1.0 F10. (protected positioning move to Z-1.0)
G65 P9811 Z-1.5 Q0.7 (single surface measurement at Z-1.5, note there is no work shift altering)
G65 P9833 (probe off)
G91 G28 Z0 (send tool home)
G90
IF[#187LT-1.501] GOTO500 (check if the tool already cut too deep and alarm)
#600=-1.5-(#187) (calculate the difference between measured and ideal depth and save in variable #600).
IF[#187GT-1.499] GOTO100 (if the tool cut too shallow, go to the beginning of the program and recut)
GOTO501
N500 #3000=100(Tool cut too deep)
N501
M99
Now we have a program that will correct for depth all by itself. If the depth is too deep, the machine will alarm, if the depth is too shallow, the probe will measure the difference and re-machine to depth all without operator intervention or human error Automation is a beautiful thing.
Macro programming is one way Amco Manufacturing increases efficiency. Contact us to see how we can help you manufacture your machined parts.